How to Display Mastodon Toots in a Linux Terminal
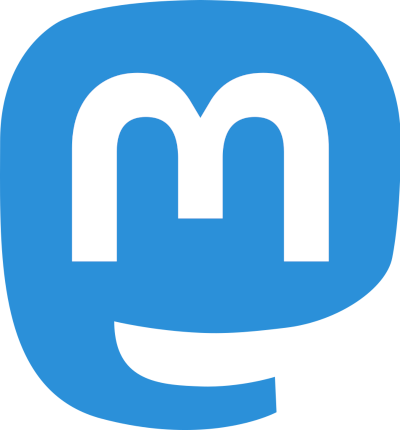
If you’re a Mastodon user and want to keep track of your home timeline directly from your Linux terminal, this simple script can help you achieve just that. With a few lines of Bash code and the power of curl
and jq
, you’ll be able to fetch and display the latest toots from your Mastodon instance without leaving the command line.
Prerequisites:
- A Mastodon account on your favorite instance.
curl
andjq
installed on your Linux system.
Step 1: Create the Script..
Open a text editor and paste in the code below.
#!/bin/env bash
INSTANCE="https://fosstodon.org" # Replace with your Mastodon instance URL
# Function to fetch and display the timeline
function display_timeline {
# Fetch the public timeline using curl and the Mastodon API
toot_timeline=$(curl -s "$INSTANCE/api/v1/timelines/public" | jq -r '.[] | .content? | gsub("<[^>]*>"; "")' | head -n 20)
# Loop through the toot texts and display them
while IFS= read -r toot_text; do
# If the toot text is empty or only contains spaces, continue to the next toot
if [ -z "${toot_text// }" ]; then
continue
fi
# Print the cleaned text-only toot content with a blank line after each toot
echo "$toot_text"
echo
done <<< "$toot_timeline"
}
# Display the timeline initially
display_timeline
# Loop to check for new toots
while true; do
sleep 60 # Wait for 60 seconds before fetching the timeline again
clear # Clear the terminal screen
# Display the timeline and check for new toots
display_timeline
done
Step 2: Customize & saving the Script..
Replace https://your-mastodon-instance
with the URL for your Mastodon instance & then save the script as toot.sh
.
Step 3: Make the script executable & then run it..
The first line makes the script executable & the second line runs the script.
chmod +x toot.sh
./toot.sh
The script will start displaying the latest public toots from your Mastodon instance. It will refresh every 60 seconds, displaying any new toots that appear in the public timeline. That’s it! You’ve now successfully set up a simple script to display Mastodon toots in your Linux terminal. Happy tooting !!
With just a few lines of code, you can now keep track of the latest toots on your Mastodon instance right from your Linux terminal. It may not be pretty, and it may not even be the best solution, but HEY.. it works !! Enjoy the convenience of staying updated with your Mastodon timeline without ever leaving the command line.
GIST LINK
PLEASE NOTE: For security reasons, avoid using your Mastodon credentials in the script. Instead, rely on public timelines or use access tokens for authentication.